Bootstrap Accordion
In this tutorial you will learn how to create accordion with Bootstrap.
Creating Accordion Widget with Bootstrap
Accordion menus and widgets are widely used on the websites to manage the large amount of content and navigation lists. With Bootstrap collapse plugin you can either create accordion or a simple collapsible panel without writing any JavaScript code.
The following example will show you how to build a simple accordion widget using the Bootstrap collapsible plugin and the panel component.
Example
Try this code »<div class="accordion" id="myAccordion"> <div class="card"> <div class="card-header" id="headingOne"> <h2 class="mb-0"> <button type="button" class="btn btn-link" data-toggle="collapse" data-target="#collapseOne">1. What is HTML?</button> </h2> </div> <div id="collapseOne" class="collapse" aria-labelledby="headingOne" data-parent="#myAccordion"> <div class="card-body"> <p>HTML stands for HyperText Markup Language. HTML is the standard markup language for describing the structure of web pages. <a href="https://www.tutorialrepublic.com/html-tutorial/" target="_blank">Learn more.</a></p> </div> </div> </div> <div class="card"> <div class="card-header" id="headingTwo"> <h2 class="mb-0"> <button type="button" class="btn btn-link collapsed" data-toggle="collapse" data-target="#collapseTwo">2. What is Bootstrap?</button> </h2> </div> <div id="collapseTwo" class="collapse show" aria-labelledby="headingTwo" data-parent="#myAccordion"> <div class="card-body"> <p>Bootstrap is a sleek, intuitive, and powerful front-end framework for faster and easier web development. It is a collection of CSS and HTML conventions. <a href="https://www.tutorialrepublic.com/twitter-bootstrap-tutorial/" target="_blank">Learn more.</a></p> </div> </div> </div> <div class="card"> <div class="card-header" id="headingThree"> <h2 class="mb-0"> <button type="button" class="btn btn-link collapsed" data-toggle="collapse" data-target="#collapseThree">3. What is CSS?</button> </h2> </div> <div id="collapseThree" class="collapse" aria-labelledby="headingThree" data-parent="#myAccordion"> <div class="card-body"> <p>CSS stands for Cascading Style Sheet. CSS allows you to specify various style properties for a given HTML element such as colors, backgrounds, fonts etc. <a href="https://www.tutorialrepublic.com/css-tutorial/" target="_blank">Learn more.</a></p> </div> </div> </div> </div>
— The output of the above example will look something like this:
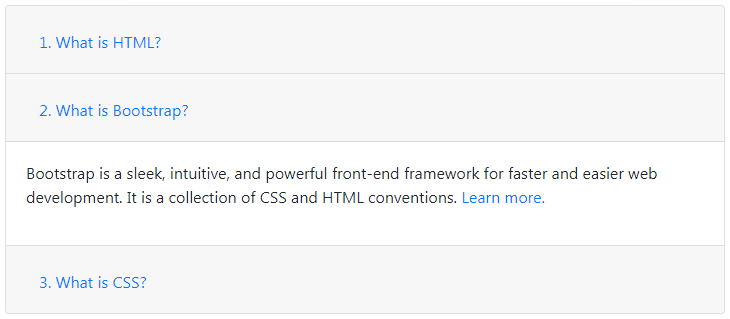
Bootstrap Accordion with Plus Minus Icons
You can also add plus minus icons to the Bootstrap accordion widget to make it visually more attractive with a few lines of jQuery code, as follow:
Example
Try this code »<script> $(document).ready(function(){ // Add minus icon for collapse element which is open by default $(".collapse.show").each(function(){ $(this).prev(".card-header").find(".fa").addClass("fa-minus").removeClass("fa-plus"); }); // Toggle plus minus icon on show hide of collapse element $(".collapse").on('show.bs.collapse', function(){ $(this).prev(".card-header").find(".fa").removeClass("fa-plus").addClass("fa-minus"); }).on('hide.bs.collapse', function(){ $(this).prev(".card-header").find(".fa").removeClass("fa-minus").addClass("fa-plus"); }); }); </script>
— The output of the above example will look something like this:
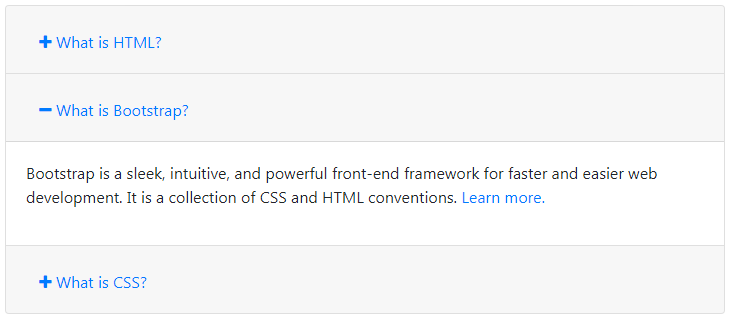
Similarly, you can add arrow icon to a accordion utilizing the jQuery and CSS transition effect.
The show.bs.collapse and hide.bs.collapse in the example above are collapse events, you'll learn about the events little later in this chapter.
Expanding and Collapsing Elements via Data Attributes
You can use the Bootstrap collapse feature for expanding and collapsing any specific element via data attributes without using the accordion markup.
Example
Try this code »<!-- Trigger Button HTML --> <input type="button" class="btn btn-primary" data-toggle="collapse" data-target="#toggleDemo" value="Toggle Button"> <!-- Collapsible Element HTML --> <div id="toggleDemo" class="collapse show"><p>This is a simple example of expanding and collapsing individual element via data attribute. Click on the <b>Toggle Button</b> button to see the effect.</p></div>
We've just created a collapsible control without writing any JavaScript code. Well, let's go through each part of this code one by one for a better understanding.
Explanation of Code
The Bootstrap collapse plugin basically requires the two elements to work properly — the controller element such as a button or hyperlink by clicking on which you want to collapse the other element, and the collapsible element itself.
The data-toggle="collapse" attribute (line no-2) is added to the controller element along with a attribute data-target (for buttons) or href (for anchors) to automatically assign the control of a collapsible element.
The data-target or href attribute accepts a CSS selector to apply the collapse to a specific element. Be sure to add the class .collapse to the collapsible element.
You can optionally add the class .show (line no-5) to the collapsible element in addition to the class .collapse to make it open by default.
To make the collapsible controls to work in group like accordion menu, you can utilize the Bootstrap panel component as demonstrated in the previous example.
Expanding and Collapsing Elements via JavaScript
You may also expand and collapse an individual element manually via JavaScript — just call the collapse() Bootstrap method with the id or class selector of the collapsible element in your JavaScript code.
Example
Try this code »<!-- JavaScript to Expand and Collapse Element --> <script> $(document).ready(function(){ $(".btn").click(function(){ $("#toggleDemo").collapse('toggle'); }); }); </script> <!-- Trigger Button HTML --> <input type="button" class="btn btn-primary" value="Toggle Button"> <!-- Collapsible Element HTML --> <div id="toggleDemo" class="collapse show"><p>This is a simple example of expanding and collapsing individual element via JavaScript. Click on the <b>Simple Collapsible</b> button to see the effect.</p></div>
Options
There are certain options which may be passed to collapse() Bootstrap method to customize the functionality of a collapsible element.
Name
Type
Default Value
Description
parent
selector
false
All other collapsible elements under the specified parent will be closed while this collapsible item is shown on invocation.
toggle
boolean
true
Toggles the collapsible element on invocation.
You can also set these options using the data attributes on accordion — just append the option name to data-, like data-parent="#myAccordion", data-toggle="false" etc. as demonstrated in the basic implementation.
Methods
These are the standard bootstrap's collapse methods:
.collapse(options)
This method activates your content as a collapsible element.
Example
Try this code »<script> $(document).ready(function(){ $(".btn").click(function(){ $("#myCollapsible").collapse({ toggle: false }); }); }); </script>
.collapse('toggle')
This method toggles (show or hide) a collapsible element.
Example
Try this code »<script> $(document).ready(function(){ $(".toggle-btn").click(function(){ $("#myCollapsible").collapse('toggle'); }); }); </script>
.collapse('show')
This method shows a collapsible element.
Example
Try this code »<script> $(document).ready(function(){ $(".show-btn").click(function(){ $("#myCollapsible").collapse('show'); }); }); </script>
.collapse('hide')
This method hides a collapsible element.
Example
Try this code »<script> $(document).ready(function(){ $(".hide-btn").click(function(){ $("#myCollapsible").collapse('hide'); }); }); </script>
Events
Bootstrap's collapse class includes few events for hooking into collapse functionality.
Event
Description
show.bs.collapse
This event fires immediately when the show instance method is called.
shown.bs.collapse
This event is fired when a collapse element has been made visible to the user. It will wait until the CSS transition process has been fully completed before getting fired.
hide.bs.collapse
This event is fired immediately when the hide method has been called.
hidden.bs.collapse
This event is fired when a collapse element has been hidden from the user. It will wait until the CSS transition process has been fully completed before getting fired.
The following example displays an alert message to the user when sliding transition of a collapsible element has been fully completed.
Example
Try this code »<script> $(document).ready(function(){ $("#myCollapsible").on('hidden.bs.collapse', function(){ alert("Collapsible element has been completely closed."); }); }); </script>
0 Comments