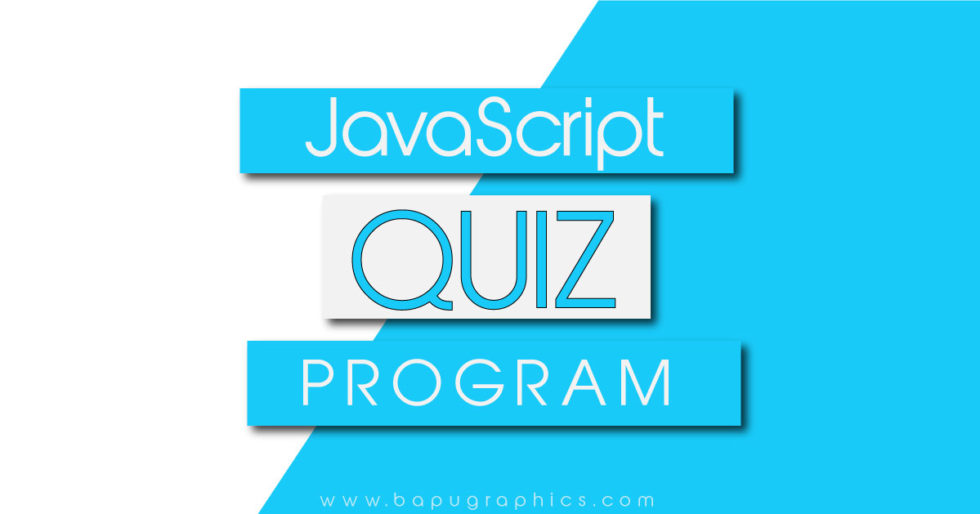
JavaScript Quiz Program: We see many quizzes online and maybe play it every day. Because quiz is a way to test and improve your skills. Creating a JavaScript quiz is a cool learning exercise. It teaches you how to use events, manipulate the DOM, handle user input. In this article you will learn to create a javascript quiz app for your basic needs after that, you can use it on your website.
Today you will learn to create a similar quiz program with javascript. Previously I had already shared how to create a Quiz program in PHP, Now its time for JavaScript version. In this program, you can add a new question answer. If you have basic knowledge of JavaScript then you can understand the whole program easily. In this program, maybe 60-70% functions made in javascript & left other parts are in HTML & CSS. <h1> title and frame are built on HTML, style in CSS, left everything is built on JavaScript. Even, Question & Answers in JavaScript file.
Preview Of JavaScript Quiz App:
Here’s a look at how this program will look like after develop.
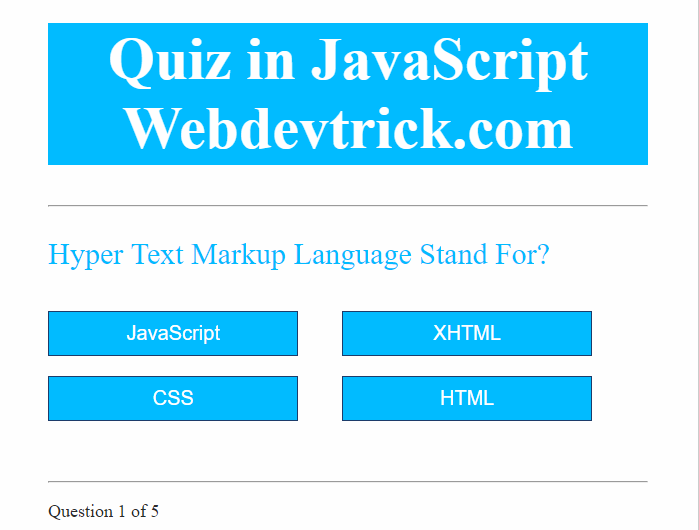
You May Also Like:
Registration Form with Validation Example and Source Code
Basic JavaScript Calculator Source Code | HTML, CSS
JavaScript Quiz Program Source Code
I had created 3 files for this program first one is for HTML file, the Second one is for CSS file, & the third one is for JavaScript ( JS ) file. I had put comments where you can add or change question answers in the JS file. You can change layout style in CSS file provided in this program.
Now create 3 files & follow the steps I have given below. File names are:
index.html
style.css
question.js
Index.html
Paste these codes are five below in index.html file you have created.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
<!DOCTYPE html>
<!-- code by webdevtrick (https://webdevtrick.com) -->
<html>
<head lang="en">
<meta charset="UTF-8">
<title>Quiz</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="grid">
<div id="quiz">
<h1>Quiz in JavaScript Webdevtrick.com</h1>
<hr style="margin-bottom: 20px">
<p id="question"></p>
<div class="buttons">
<button id="btn0"><span id="choice0"></span></button>
<button id="btn1"><span id="choice1"></span></button>
<button id="btn2"><span id="choice2"></span></button>
<button id="btn3"><span id="choice3"></span></button>
</div>
<hr style="margin-top: 50px">
<footer>
<p id="progress">Question x of y</p>
</footer>
</div>
</div>
<script src="question.js"></script>
</body>
</html>
style.css
Now, pastes these codes in style.css file you have created before.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
/** code by webdevtrick (https://webdevtrick.com) **/
body {
background-color: #413D3D;
}
.grid {
width: 600px;
height: 500px;
margin: 0 auto;
background-color: #fff;
padding: 10px 50px 50px 50px;
border: 2px solid #cbcbcb;
}
.grid h1 {
font-family: "sans-serif";
background-color: #01BBFF;
font-size: 60px;
text-align: center;
color: #ffffff;
padding: 2px 0px;
}
#score {
color: #01BBFF;
text-align: center;
font-size: 30px;
}
.grid #question {
font-family: "monospace";
font-size: 30px;
color: #01BBFF;
}
.buttons {
margin-top: 30px;
}
#btn0, #btn1, #btn2, #btn3 {
background-color: #01BBFF;
width: 250px;
font-size: 20px;
color: #fff;
border: 1px solid #1D3C6A;
margin: 10px 40px 10px 0px;
padding: 10px 10px;
}
#btn0:hover, #btn1:hover, #btn2:hover, #btn3:hover {
cursor: pointer;
background-color: #01BBFF;
}
#btn0:focus, #btn1:focus, #btn2:focus, #btn3:focus {
outline: 0;
}
#progress {
color: #2b2b2b;
font-size: 18px;
}
question.js
Finally, paste these following codes in question.js file.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
// code by webdevtrick (https://webdevtrick.com)
function Quiz(questions) {
this.score = 0;
this.questions = questions;
this.questionIndex = 0;
}
Quiz.prototype.getQuestionIndex = function() {
return this.questions[this.questionIndex];
}
Quiz.prototype.guess = function(answer) {
if(this.getQuestionIndex().isCorrectAnswer(answer)) {
this.score++;
}
this.questionIndex++;
}
Quiz.prototype.isEnded = function() {
return this.questionIndex === this.questions.length;
}
function Question(text, choices, answer) {
this.text = text;
this.choices = choices;
this.answer = answer;
}
Question.prototype.isCorrectAnswer = function(choice) {
return this.answer === choice;
}
function populate() {
if(quiz.isEnded()) {
showScores();
}
else {
// show question
var element = document.getElementById("question");
element.innerHTML = quiz.getQuestionIndex().text;
// show options
var choices = quiz.getQuestionIndex().choices;
for(var i = 0; i < choices.length; i++) {
var element = document.getElementById("choice" + i);
element.innerHTML = choices[i];
guess("btn" + i, choices[i]);
}
showProgress();
}
};
function guess(id, guess) {
var button = document.getElementById(id);
button.onclick = function() {
quiz.guess(guess);
populate();
}
};
function showProgress() {
var currentQuestionNumber = quiz.questionIndex + 1;
var element = document.getElementById("progress");
element.innerHTML = "Question " + currentQuestionNumber + " of " + quiz.questions.length;
};
function showScores() {
var gameOverHTML = "<h1>Result</h1>";
gameOverHTML += "<h2 id='score'> Your scores: " + quiz.score + "</h2>";
var element = document.getElementById("quiz");
element.innerHTML = gameOverHTML;
};
// create questions here
var questions = [
new Question("Hyper Text Markup Language Stand For?", ["JavaScript", "XHTML","CSS", "HTML"], "HTML"),
new Question("Which language is used for styling web pages?", ["HTML", "JQuery", "CSS", "XML"], "CSS"),
new Question("Which is not a JavaScript Framework?", ["Python Script", "JQuery","Django", "NodeJS"], "Django"),
new Question("Which is used for Connect To Database?", ["PHP", "HTML", "JS", "All"], "PHP"),
new Question("Webdevtrick.com is about..", ["Web Design", "Graphic Design", "SEO & Development", "All"], "All")
];
// create quiz
var quiz = new Quiz(questions);
// display quiz
populate();
That’s It. Now you’ve successfully created a quiz program in JavaScript. If you have any doubt comment down below. For more article/source code like this visit this blog regularly.
0 Comments